Introduction to QuickAccess
NOTE
QuickAccess information is never encrypted.
This allows you to save certain attributes separately from the game, so that they are available without having to load the entire file. It is used for the menu that lists the saved games.
You can save all kinds of information and this is available in the menu instantly as it is not required to load all the information in the file.
The types of data that Quick Access supports at the moment are: bool, char, double, float, int, long, short, sbyte, uint, ulong, ushort, string, byte, byte[]
.
Example of how to save and read QuickAccess
In this example we will show how to save a field, kills, this could be any other field that you want to show in the saved menu.
You will need to have a Component where you will store the information you want to save:
using UnityEngine;
using SaveIsEasy;
[RequireComponent(typeof(SaveIsEasyComponent))]
public class QuickAccessExample : MonoBehaviour {
[QuickAccess]
public int Kills;
}
After adding this component to a GameObject, Add the "SaveIsEasyComponent" component in it you have to select the kills field of the component to be saved.
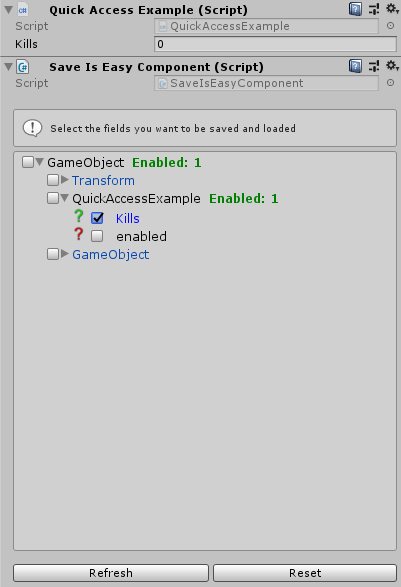
Now every time you save a game that has this GameObject you will save as QuickAccess the information of kills.
You can read it in the following example, assuming that the game was saved with the name "DefaultLevelName":
using UnityEngine;
using SaveIsEasy;
public class ReadQuickAccess : MonoBehaviour {
void Start() {
SceneFile file = SaveIsEasyAPI.GetSceneFile("DefaultLevelName");
if(file == null) {
Debug.LogError("The saved was not found!");
return;
}
//Let it be noted that Kills, K is capitalized. the function distinguishes between uppercase and lowercase.
int kills = file.GetAccessObjectAsType<int>("Kills");
Debug.Log("Quick Access Kills: " + kills);
}
}